What if you could peek into the future of Tesla’s stock price with just a few lines of Python? I’ve built an algorithm that tries to do exactly that—predicting TSLA’s price over the next 1-2 weeks using free data and a sprinkle of machine learning. It’s not a magic wand (stocks are wild), but it’s a fun, hands-on way to explore stock prediction. Want to know how it works, why Tesla’s a perfect guinea pig, and how you can try it yourself? Let’s dive in.
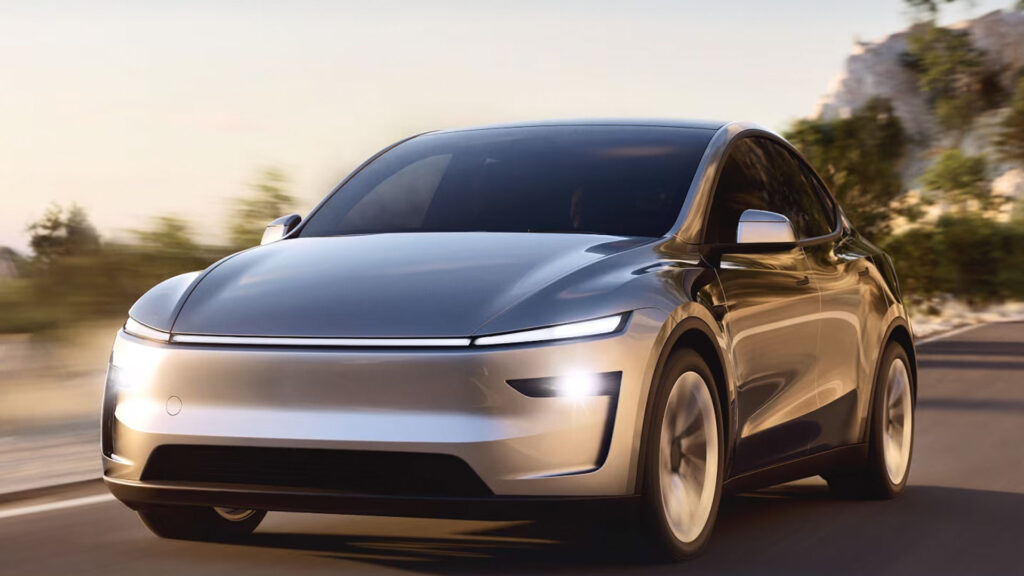
Why Tesla? The Stock Market’s Wild Child
Tesla (TSLA) isn’t your average stock. One day it’s soaring on a new factory announcement, the next it’s dipping because Elon Musk tweeted something cryptic. In 2024 alone, TSLA has swung from $180 to over $300—sometimes in a single month! That volatility makes it a thrilling challenge for prediction. Could a Python script tame this beast? I had to find out.
The Algorithm: A Deep Dive into How It Works
This isn’t some black-box Wall Street tool—it’s a DIY Python script you can run on your laptop. Here’s the step-by-step breakdown of how it predicts Tesla’s stock price:
- Fetching Free Data with yfinance
I use the yfinance library to grab Tesla’s historical stock data from Yahoo Finance. It’s free, no sign-up required, and gives me daily prices (open, high, low, close) going back a year. For this run, I pulled data from January 2024 to April 3, 2025 (today’s date). That’s about 250 trading days—enough to spot trends without overloading my model. - Crafting Features to Spot Patterns
Raw prices alone won’t cut it. I add two key indicators:- 5-day Moving Average (MA5): Averages the last 5 days’ closing prices to smooth out random blips.
- 20-day Moving Average (MA20): Looks at 20 days for a broader trend.
These are like the stock’s heartbeat—short-term pulses and longer-term rhythms. The algorithm uses them to guess where the price might head next.
- Training with Linear Regression
I picked Linear Regression from scikit-learn as my model. It’s simple but powerful: it studies how past prices and moving averages link to the next day’s price, then draws a “best-fit” line through the data. For example, if TSLA’s been climbing steadily, it’ll lean toward an upward prediction. I train it on all but the last day, then let it predict forward. - Forecasting 14 Days, One Step at a Time
Starting with the latest data (say, April 3’s close), it predicts April 4’s price. Then it updates the moving averages with that guess and predicts April 5. It repeats this 14 times, building a two-week forecast. But here’s where I hit a snag—my first version was too smooth, creeping up $0.10 a day. Stocks don’t do that! - Injecting Volatility for Realism
Tesla’s daily swings are more like $5-$10, not pennies. So I calculated its historical volatility—how much its price jumps day-to-day (using percentage changes)—and added random noise to each prediction. Think of it like weather: the model sets the trend (sunny or stormy), but the noise adds gusts and showers. Now it feels more like a real stock. - Plotting the Future
Using matplotlib, I graph the historical prices in blue and the 14-day forecast in red. It’s not just numbers—you can see where Tesla’s been and where it might go. Visuals make it click.
A Real Prediction: What It Looks Like
Here’s a sample run from April 3, 2025:
- Predicted Tesla Stock Prices for the Next 14 Days:
- 2025-04-04: $259.46
- 2025-04-05: $254.17
- 2025-04-06: $254.11
- 2025-04-07: $248.88
- 2025-04-08: $244.45
- …up to April 17: $246..81
Let’s be honest—predicting stocks is like predicting the weather in a hurricane. Linear Regression assumes a steady trend, but TSLA doesn’t play nice. A new Cybertruck reveal or a market crash could flip the script overnight. My first version was so stable it felt fake; adding volatility helped, but it’s still a toy compared to Wall Street’s AI beasts. For real accuracy, you’d need:
- More Data: Trading volume, news sentiment, even Elon’s X posts.
- Better Models: LSTM neural networks or ARIMA could catch wilder swings.
Why This Matters (Even If It’s Not Perfect)
So why bother? Because it’s a gateway. This script taught me how data, math, and code can wrestle with a chaotic market. It’s not about getting rich—it’s about understanding. Plus, Tesla’s a hot topic. People search “Tesla stock prediction” all the time—maybe they’ll stumble on this and stick around.
3 Ways to Make It Your Own
Want to try it? Here’s how to level up:
- Tweak the Volatility: Multiply the noise by 2 for bigger swings—or 0.5 for calmer ones. See what fits TSLA’s vibe.
- Swap the Stock: Change “TSLA” to “AAPL” (Apple) or “GME” (GameStop) in the code. Compare their rollercoasters!
- Add News: Scrape X for Tesla buzz (with an API like tweepy) and weigh it in. Bullish tweets might nudge the price up.
Get the Code and Join the Fun
You can grab the full script [link to your GitHub/repo or paste it below if you prefer]. Install these Python libraries:
bash
pip install yfinance pandas numpy scikit-learn matplotlib
import yfinance as yf
import pandas as pd
import numpy as np
from sklearn.linear_model import LinearRegression
from datetime import datetime, timedelta
import matplotlib.pyplot as plt
# Fetch Tesla stock data
def fetch_tesla_data():
ticker = "TSLA"
stock = yf.download(ticker, start="2024-01-01", end=datetime.today().strftime('%Y-%m-%d'))
return stock
# Prepare the data
def prepare_data(df):
df = df[['Close']].copy()
df['MA5'] = df['Close'].rolling(window=5).mean()
df['MA20'] = df['Close'].rolling(window=20).mean()
df = df.dropna()
df['Target'] = df['Close'].shift(-1)
df = df[:-1]
# Calculate historical daily volatility (standard deviation of daily returns)
df['Returns'] = df['Close'].pct_change()
volatility = df['Returns'].std()
return df, volatility
# Train the model
def train_model(df):
X = df[['Close', 'MA5', 'MA20']]
y = df['Target']
model = LinearRegression()
model.fit(X, y)
return model
# Predict with added volatility
def predict_next_14_days(model, df, volatility):
last_data = df.tail(1)[['Close', 'MA5', 'MA20']].values[0]
predictions = []
future_dates = []
for i in range(14):
next_price = model.predict([last_data])[0]
# Add random noise based on historical volatility
noise = np.random.normal(0, volatility * last_data[0]) # Scale by current price
next_price += noise
predictions.append(max(next_price, 0)) # Ensure price doesn’t go negative
# Update features
last_data = [
next_price,
(last_data[0] * 4 + next_price) / 5,
(last_data[1] * 19 + next_price) / 20
]
future_dates.append(datetime.today() + timedelta(days=i + 1))
return future_dates, predictions
# Plot results
def plot_results(historical_df, future_dates, predictions):
plt.figure(figsize=(12, 6))
plt.plot(historical_df.index, historical_df['Close'], label='Historical Close', color='blue')
plt.plot(future_dates, predictions, label='Predicted Close (Next 14 Days)', color='red', linestyle='--')
plt.title('Tesla Stock Price Prediction (Next 1-2 Weeks)')
plt.xlabel('Date')
plt.ylabel('Price (USD)')
plt.legend()
plt.grid()
plt.show()
# Main execution
if __name__ == "__main__":
tesla_data = fetch_tesla_data()
prepared_data, volatility = prepare_data(tesla_data)
model = train_model(prepared_data)
future_dates, predictions = predict_next_14_days(model, prepared_data, volatility)
print("Predicted Tesla Stock Prices for the Next 14 Days:")
for date, price in zip(future_dates, predictions):
print(f"{date.strftime('%Y-%m-%d')}: ${price:.2f}")
plot_results(tesla_data, future_dates, predictions)
Predicted Tesla Stock Prices for the Next 14 Days:
2025-04-04: $259.46
2025-04-05: $254.17
2025-04-06: $254.11
2025-04-07: $248.88
2025-04-08: $244.45
2025-04-09: $250.94
2025-04-10: $252.81
2025-04-11: $255.14
2025-04-12: $257.77
2025-04-13: $243.30
2025-04-14: $231.13
2025-04-15: $233.53
2025-04-16: $235.81
2025-04-17: $246.81
Run it, tweak it, break it—make it yours. My blog’s view rate was lagging, so I’m sharing this to spark some buzz. Can Python outsmart the market? Probably not—but it’s a blast to try. What’s your prediction for TSLA? Drop it in the comments—I’ll check back and compare notes!