“Want to predict Tesla’s stock price for the next 14 business days? I’ve built a Python script using linear regression, moving averages, and historical volatility—excluding weekends and U.S. holidays! Here’s how it works:
- Fetches TSLA data with yfinance
- Trains a model on closing prices + 5-day/20-day MAs
- Predicts 14 business days ahead, skipping Saturdays, Sundays, and holidays
- Adds realistic noise based on past volatility
Check out the code below and try it yourself! (You’ll need yfinance, pandas, numpy, sklearn, matplotlib, and holidays installed.) Feedback welcome!”
python
import yfinance as yf
import pandas as pd
import numpy as np
from sklearn.linear_model import LinearRegression
from datetime import datetime, timedelta
import matplotlib.pyplot as plt
import holidays
us_holidays = holidays.US()
def fetch_tesla_data():
ticker = "TSLA"
stock = yf.download(ticker, start="2024-01-01", end=datetime.today().strftime('%Y-%m-%d'))
return stock
def prepare_data(df):
df = df[['Close']].copy()
df['MA5'] = df['Close'].rolling(window=5).mean()
df['MA20'] = df['Close'].rolling(window=20).mean()
df = df.dropna()
df['Target'] = df['Close'].shift(-1)
df = df[:-1]
df['Returns'] = df['Close'].pct_change()
volatility = df['Returns'].std()
return df, volatility
def train_model(df):
X = df[['Close', 'MA5', 'MA20']]
y = df['Target']
model = LinearRegression()
model.fit(X, y)
return model
def is_business_day(date):
return date.weekday() < 5 and date not in us_holidays
def predict_next_14_business_days(model, df, volatility):
last_data = df.tail(1)[['Close', 'MA5', 'MA20']].values[0]
predictions = []
future_dates = []
days_ahead = 0
business_days_count = 0
while business_days_count < 14:
next_date = datetime.today() + timedelta(days=days_ahead + 1)
if is_business_day(next_date):
next_price = model.predict([last_data])[0]
noise = np.random.normal(0, volatility * last_data[0])
next_price += noise
predictions.append(max(next_price, 0))
future_dates.append(next_date)
last_data = [
next_price,
(last_data[0] * 4 + next_price) / 5,
(last_data[1] * 19 + next_price) / 20
]
business_days_count += 1
days_ahead += 1
return future_dates, predictions
def plot_results(historical_df, future_dates, predictions):
plt.figure(figsize=(12, 6))
plt.plot(historical_df.index, historical_df['Close'], label='Historical Close', color='blue')
plt.plot(future_dates, predictions, label='Predicted Close (Next 14 Business Days)', color='red', linestyle='--')
plt.title('Tesla Stock Price Prediction (Next 14 Business Days)')
plt.xlabel('Date')
plt.ylabel('Price (USD)')
plt.legend()
plt.grid()
plt.show()
if __name__ == "__main__":
tesla_data = fetch_tesla_data()
prepared_data, volatility = prepare_data(tesla_data)
model = train_model(prepared_data)
future_dates, predictions = predict_next_14_business_days(model, prepared_data, volatility)
print("Predicted Tesla Stock Prices for the Next 14 Business Days:")
for date, price in zip(future_dates, predictions):
print(f"{date.strftime('%Y-%m-%d')}: ${price:.2f}")
plot_results(tesla_data, future_dates, predictions)
“Run it and see where TSLA might head—happy coding and trading! #Python #StockPrediction #Tesla”
Output Result:
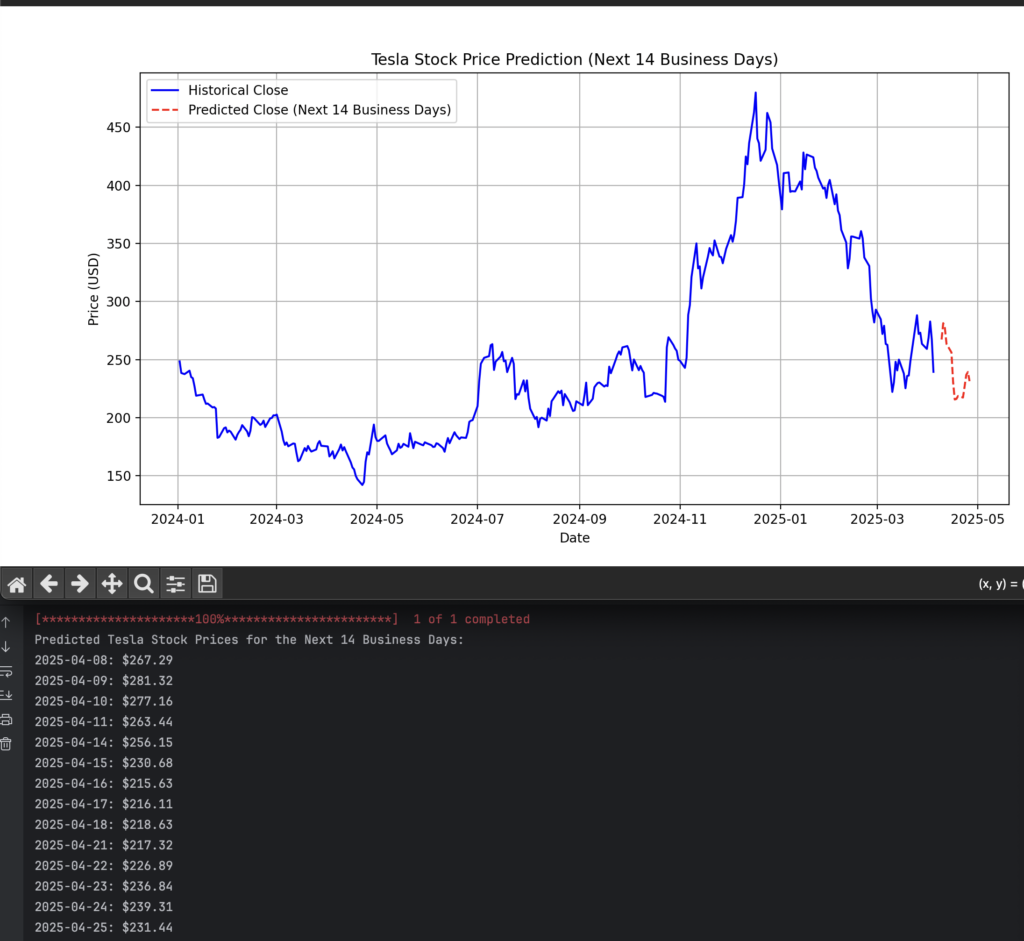
As of the stock price today:
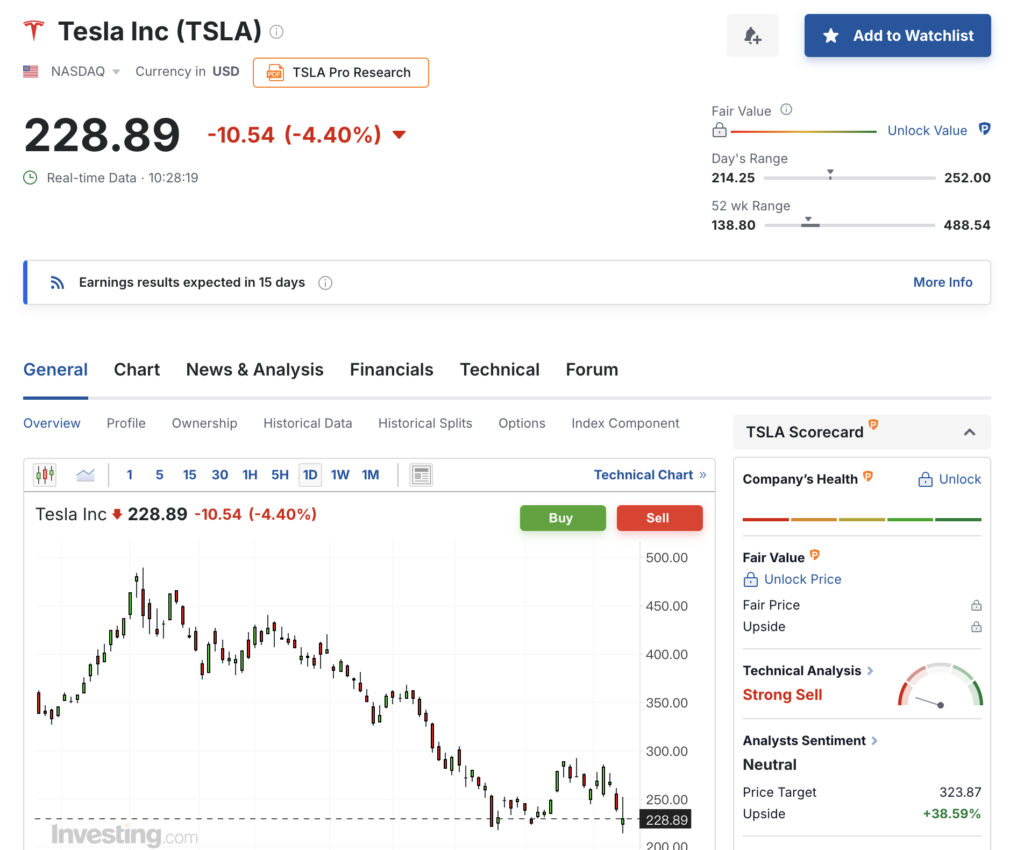